Event Listeners
Event Listeners are used to add and remove JavaScript Events. The most preferred and recommended way of dealing with javascript events is to use event listeners. These event listeners were introduced in ECMA 5. Thus all modern web browsers support them (Except I.E 8 and below).
To use event listeners, we use addEventListener() function (to add event), and removeEventListener() function (to remove an event).
Advantage of using Event Listeners
- Can add multiple functions on same event.
- Event can be removed using removeEventListener function.
- Event Propagation can be changed.
- Can use custom events, like touch events.
Drawback of Event Listeners
- No support for IE 8 and below.
Type of Event Listeners
addEventListener
addEventListener is the most preferred and recommended way to handle javascript events.
Inside addEventListener function, first parameter is event type string, like click, mouseover, mouseout, scroll etc and second parameter is function that will be called when event occurs. Second parameter can also be an anonymous function. Third parameter is boolean.
addEventListener with callback function
document.body.addEventListener("click",doThis);
function doThis(){
alert(this.textContent);
}
or
addEventListener with anonymous function
document.body.addEventListener("click",function(){});
In this example, we are using click event on body element. addEventListener use two parameters. First event type, and second is callback function, that is invoked when event occurs. Third parameter (Boolean value true or false) is option as it is used only when we change event propagation.
document.querySelector("button").addEventListener("click",function(){
//action
});
h3>addEventListener with named function
document.querySelector("button").addEventListener("click", myFunction);
function myFunction(){
// action
}
removeEventListener
Event listeners can be removed using removeEventListener() method. This is another advantage of using event listeners. Here is an example.
const btn=document.querySelector("button");
btn.addEventListener("click", remove);
function remove(){
alert("Clicked");
btn.removeEventListener("click",remove)
}
Event Propagation
Event Propagation is the order in which an events fire from child element to parent. By default events propagates from child to parent node, also known as bubbling.
Type of event propagations
Event Bubbling
Event Bubbling is when event fire on an elements and then bubbles up to parent Elements till it reach root node. By default all events are bubbling type. In example show, click events is used on both parent div, and child button. But button will call first.
<div id="div1">
<button id="button1">Button 1</button>
</div>
<script>
document.querySelector("#div1").addEventListener("click",function(){
console.log("you clicked div");
});
document.querySelector("#button1").addEventListener("click",function(){
console.log("you clicked button");
});
</script>
Event capturing
Event capturing is when root node is fired first, and then Propagate downwards until it reach targeted element. Capturing is possible by using third boolean parameter (true) in event listener. Here is an example.
<div id="div2">
<button id="button2">Button 1</button>
</div>
<script>
document.querySelector("#div2").addEventListener("click",function(){
alert("you clicked div");
},true);
document.querySelector("#button2").addEventListener("click",function(){
alert("you clicked button");
},true);
</script>
eventDelegation
bubbling can be used for Event Delegation. Event Delegation is the technique where we use single event to interact with multiple child elements by using event.target.
Consider a case where we have need to add events to 16 columns of single parent. Instead of using 16 click events, we can use event delegation technique for efficient work. Se example below.
const t=document.querySelector("table");
t.addEventListener("click",function(e){
if(e.target.tagName == "TD"){
e.target.classList.toggle('active');
}
});
event.stopPropagation()
event.stopPropagation() method stops propagation of event from child to parent in bubbling, or from parent to child in case of capturing.
event.stopPropagation in bubbling
event.stopPropagation() in bubbling stop propagation of event from child to parent node. In short, child event will triggered, but parent event won't.
<div id="div3">
<button id="btn3">Button</button>
</div>
<script>
document.querySelector("#div3").addEventListener("click",function(){
alert("you clicked div");
});
document.querySelector("#btn3").addEventListener("click",function(e){
alert("you clicked button");
e.stopPropagation();
});
</script>
event.stopPropagation in capturing
event.stopPropagation() in capturing stop propagation of event from parent to child node. In short, parent event will triggered, but child event won't.
<div id="div4">
<button id="btn4">Button</button>
</div>
<script>
document.querySelector("#div4").addEventListener("click",function(e){
alert("you clicked div");
e.stopPropagation();
});
document.querySelector("#btn4").addEventListener("click",function(){
alert("you clicked button");
});
</script>
event.stopImmediatePropagation()
event.stopImmediatePropagation() method stops propagation of other event listeners on same element node also.
If an element has two event listeners, and parent has one listener, stopPropagation will only stop parent event, but two listeners of element will be triggered. But in stopImmediatePropagation, only first event listener triggered. The second event listener of same element and parent event will not. See example
with event.stopPropagation
const btn=document.querySelector('button');
const div=document.querySelector('div');
btn.addEventListener("click",function(){
alert(1);
e.stopPropagation();
});
btn.addEventListener("click",function(){ alert(2)});
div.addEventListener("click",function(){ alert(3)});
with event.stopImmediatePropagation
const btn=document.querySelector('button');
const div=document.querySelector('div');
btn.addEventListener("click",function(){
alert(1);
e.stopImmediatePropagation();
});
btn.addEventListener("click",function(){ alert(2)});
div.addEventListener("click",function(){ alert(3)});
Touch Events
ES5 introduced Touch Based events in javascript . Touch events are applicable for Touch Based Devices only.
Type of Touch Events
- touchstart
- touchmove
- touchend
Touch Event Example
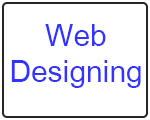
const img=document.querySelector("img);
img.addEventListener("touchstart",function(){
this.style.boxShadow="0px 0px 5px 1px #000";
});
img.addEventListener("touchmove",function(e){
e.preventDefault();
if (e.targetTouches.length == 1) {
var touch = e.targetTouches[0];
// Place element where the finger is
obj.style.left = (touch.clientX-obj.clientLeft) + 'px';
obj.style.top = (touch.clientY-obj.clientTop-350) + 'px';
// position of image should be relative in css
});
img.addEventListener("touchend",function(){
this.style.boxShadow="0px 0px 5px 1px transparent";
});
Event Listeners are not supported in IE 8 and below browser. For Old Browsers support, use property based click events, or Jquery.